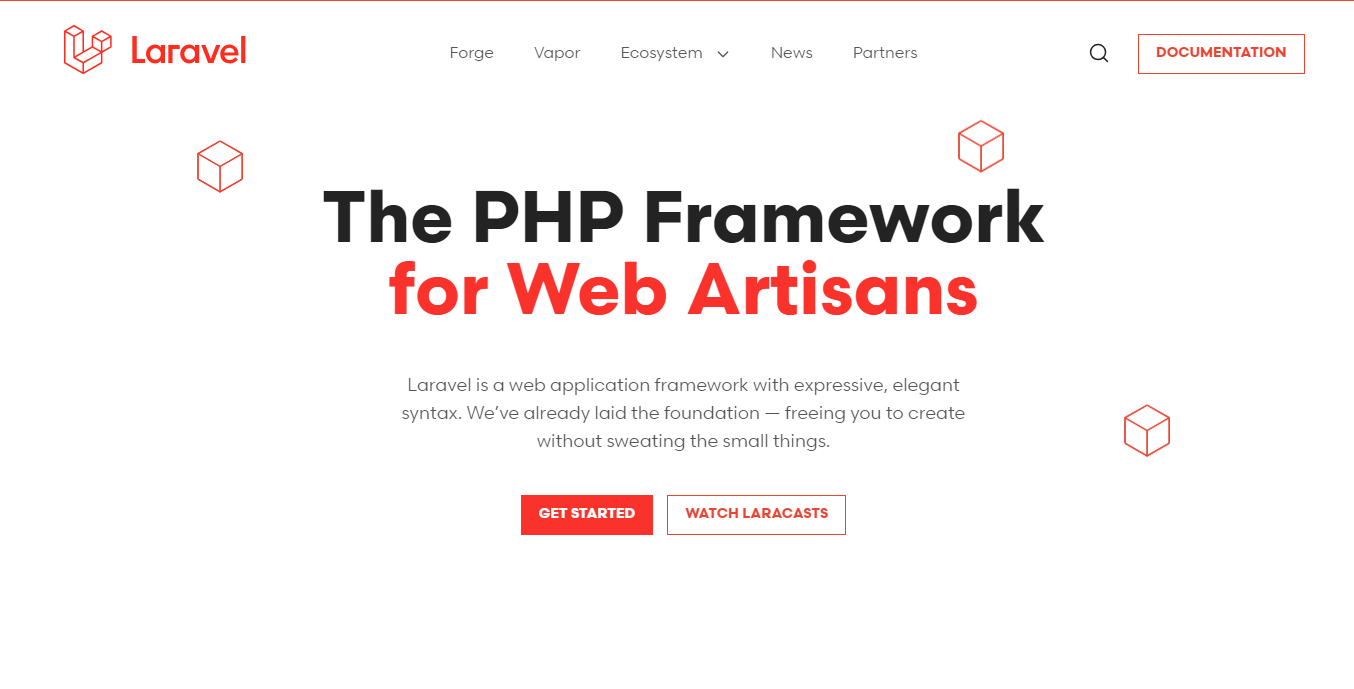
laravel localization
In this tutorial, you’ll learn how to replace a specific string with another in Laravel, utilizing a controller method and implementing the change directly in a Blade template.
Part 1: String Replacement in Laravel Controllers
Step1: Create a New Controller
- Open your Laravel project’s terminal.
- Use the
php artisan make:controller
command to create a new controller. For example, create a “StringReplaceController”:
php artisan make:controller StringReplaceController
Step2: Implement String Replacement
- Open the newly created
StringReplaceController.php
file in theapp/Http/Controllers
directory. - In a controller method, use the
str_replace()
function to replace a specific string with another:
public function replaceString($originalText)
{
$replacementText = str_replace('old_string', 'new_string', $originalText);
return view('string-replace', ['originalText' => $originalText, 'replacementText' => $replacementText]);
}
Step3: Define Route
- Open the
routes/web.php
file. - Define a route that uses the
replaceString
method in theStringReplaceController
:
Route::get('/string-replace/{text}', 'StringReplaceController@replaceString');
Part 2: String Replacement in Blade Templates
Step1: Create a Blade View
- Navigate to your Laravel project’s
resources/views
directory. - Create a new Blade view file. For example, name it “string-replace.blade.php”.
Step2: Perform String Replacement in Blade Template
- Open the “string-replace.blade.php” file.
- Inside the Blade template, use the
str_replace()
function to perform the string replacement:
<!DOCTYPE html>
<html>
<head>
<title>String Replacement Example</title>
</head>
<body>
<p>Original Text: {{ $originalText }}</p>
<p>Replaced Text: {{ str_replace('old_string', 'new_string', $replacementText) }}</p>
</body>
</html>
Conclusion
You’ve successfully learned how to replace a specific string with another using both a Laravel controller method and directly within a Blade template. This approach provides flexibility in how you implement string replacement in your Laravel application.
Feel free to explore further and apply this technique to other parts of your Laravel project where string replacement is needed. Happy coding!
Read More
1 thought on “String Replacement in Laravel Controllers and Blade Templates”
Comments are closed.