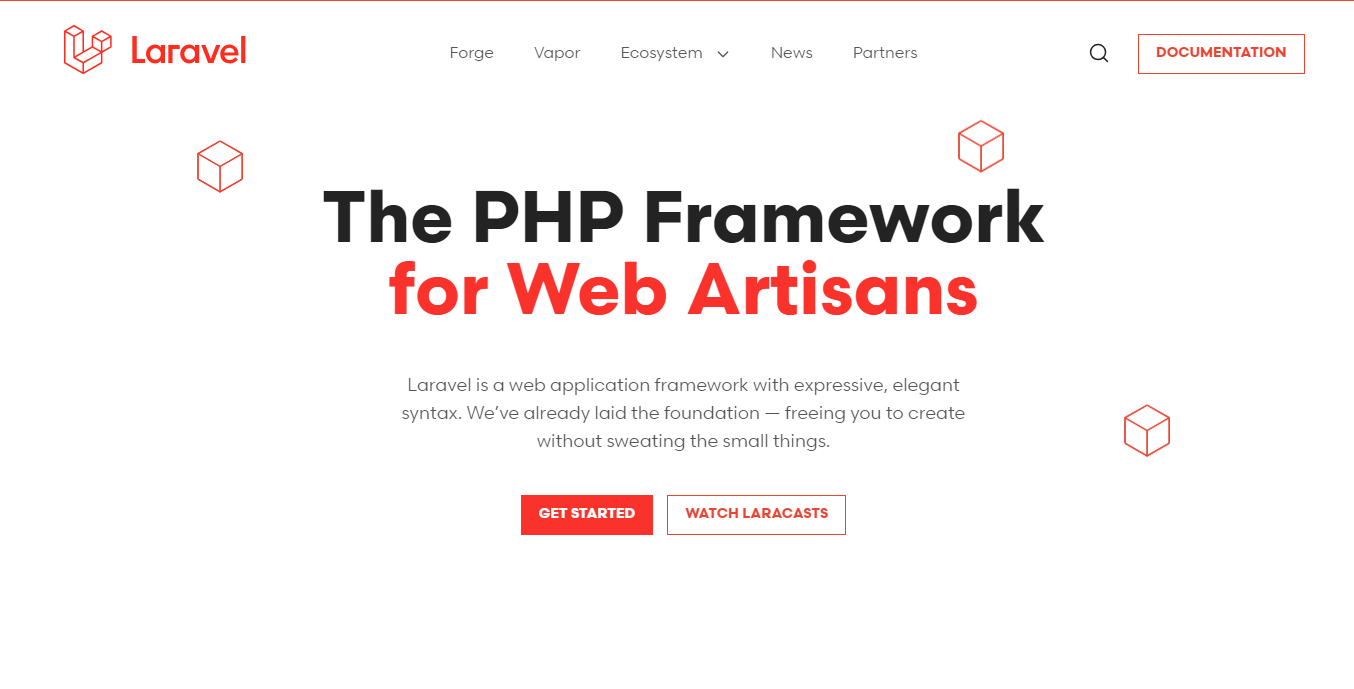
laravel localization
In this tutorial, you’ll learn two methods to capitalize the first letter of a string in Laravel: using a controller method and directly within a Blade template.
Method 1: Capitalizing First Letter in Laravel Controllers
Step1: Create a New Controller
- Open your Laravel project’s terminal.
- Use the
php artisan make:controller
command to create a new controller. For Exsample, create a “UserController”:
php artisan make:controller UserController
Step2: Capitalize First Letter in Controller
- Open the newly created
UserController.php
file in theapp/Http/Controllers
directory. - Import the
Illuminate\Support\Str
class at the top of the file:
use Illuminate\Support\Str;
- Inside a controller method, capitalize the first letter of a string using the
Str::ucfirst()
method:
public function capitalizeName($name)
{
$capitalizedName = Str::ucfirst($name);
return view('user', ['capitalizedName' => $capitalizedName]);
}
Step3: Update Route
- Open the
routes/web.php
file. - Define a route that uses the
capitalizeName
method in theUserController
:
Route::get('/user/{name}', 'UserController@capitalizeName');
Method 2: Capitalizing First Letter in Blade Templates
Step1: Create a Blade View
- Navigate to your Laravel project’s
resources/views
directory. - Create a new Blade view file and name it as “user.blade.php”.
Step2: Display Capitalized Name in Blade Template
- Open the “user.blade.php” file.
- Inside the Blade template, use the
ucfirst()
PHP function to capitalize the first letter of a variable:
<!DOCTYPE html>
<html>
<head>
<title>Capitalization Example</title>
</head>
<body>
<h1>Capitalized Name (From Controller): {{ $capitalizedName }}</h1>
<h1>Capitalized Name (Static): {{ ucfirst('john doe') }}</h1>
</body>
</html>
Conclusion
Congratulations on mastering capitalize the first letter of a string in Laravel using controller methods and Blade templates. This versatile technique enhances your ability to manipulate text throughout your Laravel applications. Explore and integrate this method to efficiently capitalize the first letter. Happy coding!
See more
2 thoughts on “Capitalize First Letter in Laravel Controllers and Blade Templates”
Comments are closed.