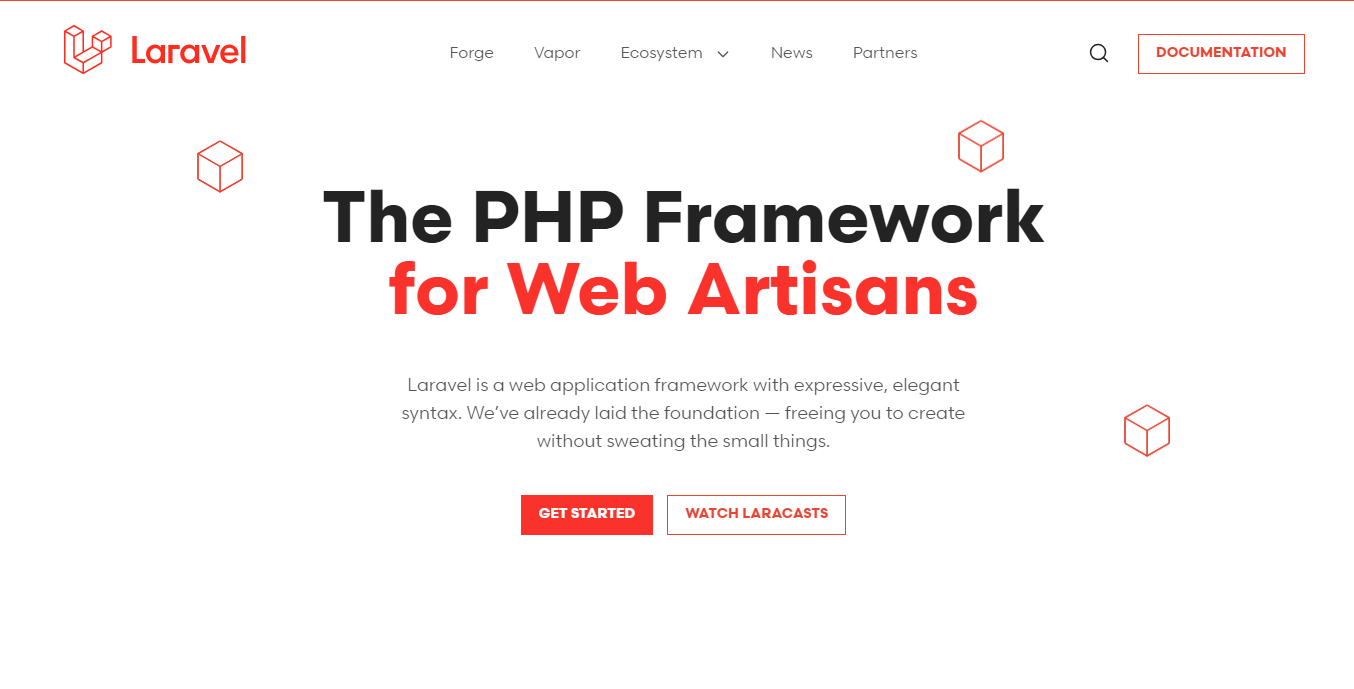
laravel localization
Enhance user experience and expand your website’s reach by implementing language localization using middleware in your Laravel project. This step-by-step guide empowers you to seamlessly switch between multiple languages, offering visitors content in their preferred language. Configure localization settings, create language-specific files, and integrate an intuitive language selector. By utilizing middleware, you ensure consistent language switching across all pages, boosting SEO and accessibility. Elevate your website’s global appeal and engage a wider audience with this robust language localization solution.
Step-by-Step Guide: Language Localization with Middleware in Laravel
- Create a New Laravel Project:
If you haven’t already, create a new Laravel project using Composer:
composer create-project --prefer-dist laravel/laravel YourProjectName
- Configure Localization:
Open config/app.php
and make sure that the locale
configuration is set to your default language (e.g., 'en'
for English).
'locale' => 'en',
- Create Language Files:
Inside the resources/lang
directory, create subdirectories for each language you want to support (e.g., en
for English, es
for Spanish). Inside each language directory, create a messages.php
file with your language-specific key-value pairs.
Create the messages.php
file for English (en
) in resources/lang/en/messages.php
:
<?php
return [
'welcome' => 'Welcome to our website!',
'about' => 'About Us',
// ... other key-value pairs
];
Create the messages.php
file for Spanish (es
) in resources/lang/es/messages.php
:
<?php
return [
'welcome' => '¡Bienvenido a nuestro sitio web!',
'about' => 'Acerca de nosotros',
// ... other key-value pairs
];
- Create Language Selector:
In your Blade view, create a language selector dropdown:
<form action="{{ route('language.change') }}" method="POST">
@csrf
<select name="locale" onchange="this.form.submit()">
<option value="en" {{ app()->getLocale() === 'en' ? 'selected' : '' }}>English</option>
<option value="es" {{ app()->getLocale() === 'es' ? 'selected' : '' }}>Español</option>
<!-- Add more language options as needed -->
</select>
</form>
- Create a Middleware:
Generate a new middleware named SetLanguage
:
php artisan make:middleware SetLanguage
Open app/Http/Middleware/SetLanguage.php
and modify the handle
method:
use Illuminate\Support\Facades\App;
use Closure;
public function handle($request, Closure $next)
{
$locale = $request->input('locale');
if (in_array($locale, ['en', 'es'])) {
App::setLocale($locale);
session(['locale' => $locale]);
}
return $next($request);
}
- Register the Middleware:
Open app/Http/Kernel.php
and add the middleware to the web
middleware group:
protected $middlewareGroups = [
'web' => [
// ...
\App\Http\Middleware\SetLanguage::class,
],
// ...
];
- Create a Route and Controller:
Define a route and controller method for handling language changes. In routes/web.php
:
use Illuminate\Support\Facades\Route;
Route::post('/language/change', 'LanguageController@changeLocale')->name('language.change');
Create a controller using the following command:
php artisan make:controller LanguageController
- Implement Controller Method:
Open app/Http/Controllers/LanguageController.php
and implement the changeLocale
method:
use Illuminate\Http\Request;
use Illuminate\Support\Facades\App;
public function changeLocale(Request $request)
{
return redirect()->back();
}
- Update Language Changing Logic:
Modify the changeLocale
method to update the locale and session:
public function changeLocale(Request $request)
{
$locale = $request->input('locale');
if (in_array($locale, ['en', 'es'])) {
App::setLocale($locale);
session(['locale' => $locale]);
}
return redirect()->back();
}
- Implement Localization in Views:
Use the trans()
function to display localized content in your Blade views:
<h1>{{ trans('messages.welcome') }}</h1>
<p>{{ trans('messages.about') }}</p>
Start your development server using php artisan serve
and visit your website.
You should be able to change the language using the dropdown selector, and the content should update accordingly.
That’s it! You’ve successfully implemented language localization using middleware in your Laravel project. This approach ensures that the language is set for each request and centralizes the language-switching logic.
Read More