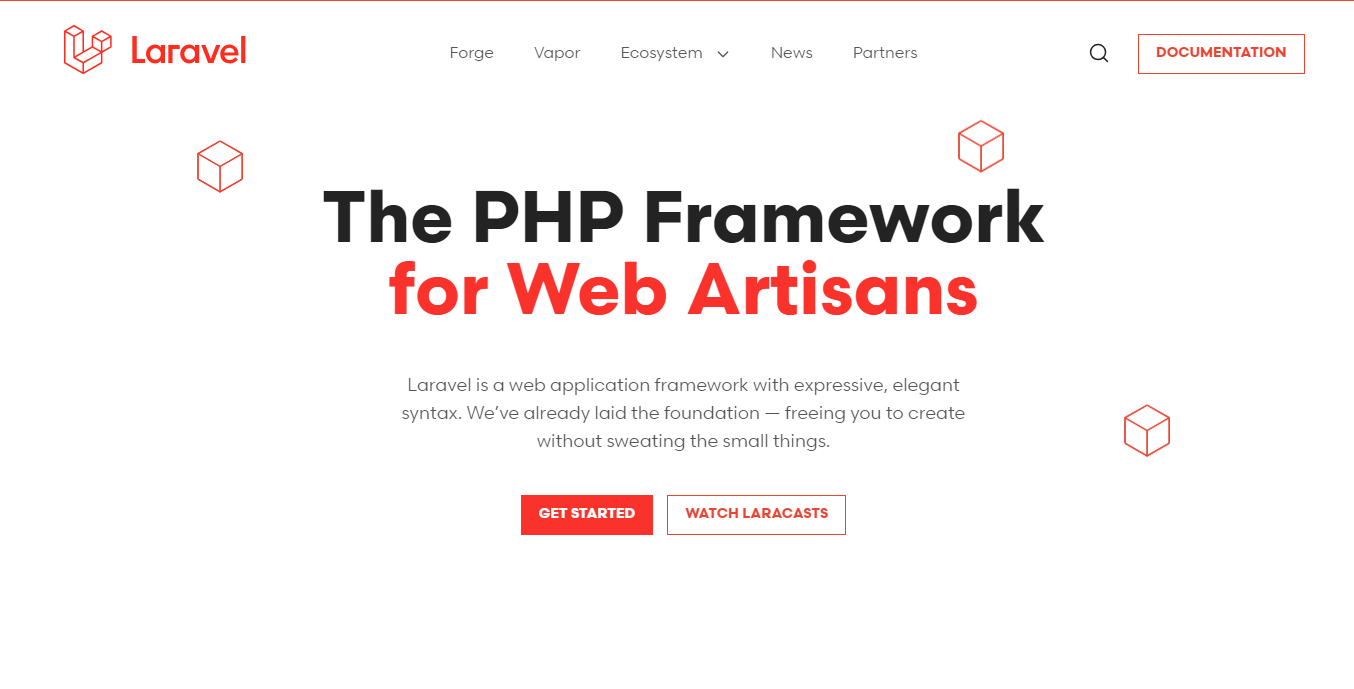
laravel localization
Laravel migrations are a powerful feature that enables developers to manage database schema changes easily. Whether you’re adding new tables, modifying existing ones, or rolling back changes, Laravel migrations streamline the process, making database management more efficient and less error-prone. In this article, we’ll cover everything you need to know about Laravel migrations, from setting them up to best practices for managing your database schema.
What Are Laravel Migrations?
Laravel migrations are version control for your database, allowing you to define and manage your database schema over time. They enable you to create, modify, and share database tables and columns in a consistent, versioned manner. Migrations are especially useful in team environments, ensuring that everyone has the same database structure.
Setting Up Migrations in Laravel
Laravel comes with built-in support for migrations, making it easy to get started. When you create a new Laravel project, the migrations
directory is automatically included in the database
directory.
php artisan make:migration create_users_table --create=users
This command creates a new migration file in the database/migrations
directory with a timestamp, ensuring that your migrations are applied in the correct order.
Creating and Running Migrations
Creating a migration is simple with Laravel’s Artisan command-line tool. Use the make:migration
command to generate a new migration file:
php artisan make:migration add_votes_to_users_table --table=users
This creates a new migration file where you can define the changes to your users
table.
Modifying Tables with Migrations
In your migration file, you’ll find two methods: up()
and down()
. The up()
method defines the changes you want to make, while the down()
method reverses those changes.
public function up()
{
Schema::table('users', function (Blueprint $table) {
$table->integer('votes')->default(0);
});
}
public function down()
{
Schema::table('users', function (Blueprint $table) {
$table->dropColumn('votes');
});
}
After defining your changes, run the migration using the following command:
php artisan migrate
Rolling Back Migrations
If you ever need to undo a migration, Laravel makes it easy with the migrate:rollback command. This will roll back the last batch of migrations:
php artisan migrate:rollback
To roll back all migrations, use:
php artisan migrate:reset
Or, to refresh your database by rolling back all migrations and then running them again:
php artisan migrate:refresh
Best Practices for Using Migrations
- Keep Migrations Small and Focused: Each migration should do one thing—create a table, add a column, etc. This makes it easier to manage and debug.
- Use Descriptive Names: Name your migrations clearly so others can understand what changes are being made.
- Backup Before Running Migrations: Always back up your database before running migrations, especially in production.
- Test Migrations Locally: Run your migrations in a local or staging environment before applying them to production to catch any issues.
Conclusion
Laravel migrations are an essential tool for managing your database schema in a structured and version-controlled way. They make it easy to collaborate with other developers, track changes, and ensure consistency across different environments. By following the best practices outlined in this guide, you can master Laravel migrations and keep your database in sync with your application code.