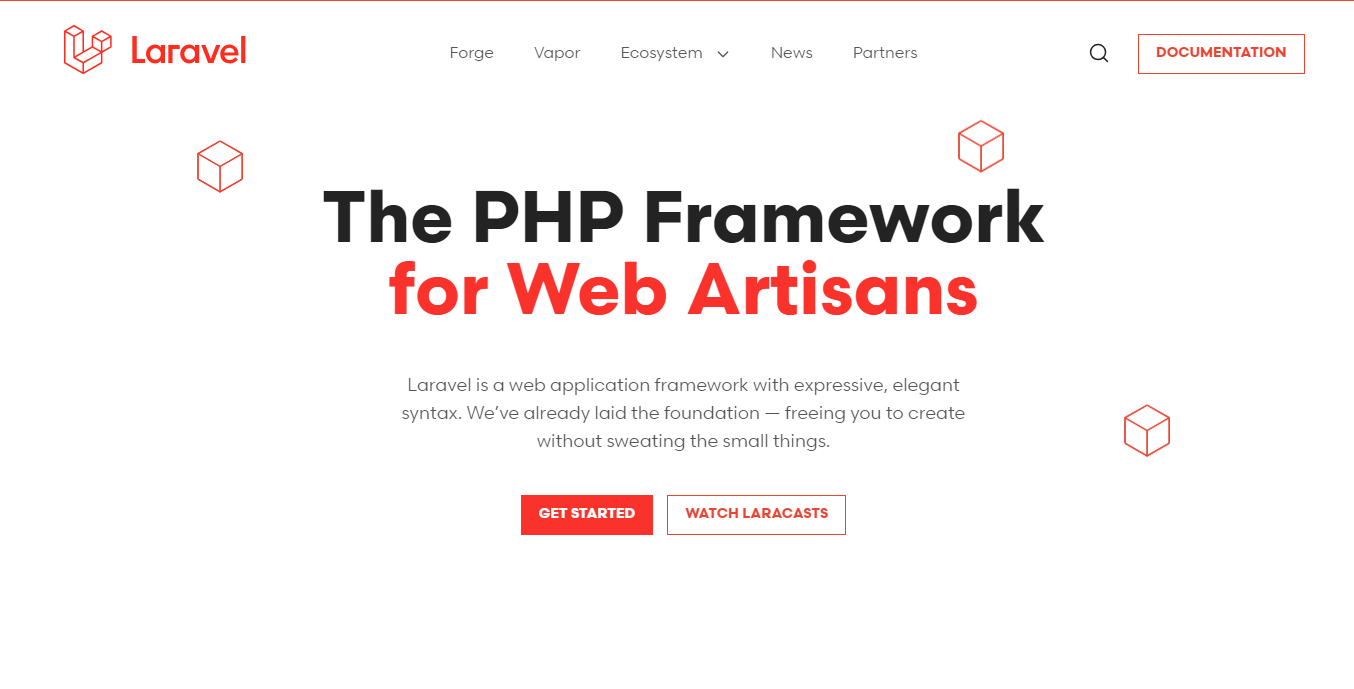
laravel localization
Efficiently schedule Zoom meetings in 2023 using new Server-to-Server OAuth or OAuth app types with Laravel. Seamlessly integrate Zoom functionality into your application
Step 1: Set up a Zoom App
- Go to the Zoom Developer Portal (https://marketplace.zoom.us) and sign in with your Zoom account or create a new account if you don’t have one.
- Click on “Build App” in the top right corner.
- Choose the app type you want to create. For scheduling meetings, select “Server-to-Server OAuth” as the app type.
- Fill in the required information for your app, such as the name, description, and company details.
- Once your app is created, you will receive a “Client ID” and “Client Secret.” Keep these credentials safe as you will need them later.
Step 2: Install Required Libraries
- Install the Guzzle HTTP client library to make HTTP requests to the Zoom API:
- Install the Guzzle HTTP client library to make HTTP requests to the Zoom API:
Step 3: Configure .env File
Add the following lines to your .env
file and fill in the values with your Zoom credentials:
ZOOM_CLIENT_ID=your_zoom_client_id
ZOOM_CLIENT_SECRET=your_zoom_client_secret
ZOOM_ACCOUNT_EMAIL=your_zoom_account_email
Step 4: Create a Controller for Zoom Meeting Scheduling
Create a new controller using the following command:
php artisan make:controller ZoomMeetingController
Step 5: Implement the Scheduling Logic in the Controller
In the ZoomMeetingController.php
file, implement the logic to schedule a Zoom meeting using cURL:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use GuzzleHttp\Client;
use App\Mail\MeetingInvitationMail;
use Illuminate\Support\Facades\Mail;
class ZoomMeetingController extends Controller
{
public function showForm()
{
return view('zoom-meeting');
}
public function scheduleMeeting(Request $request)
{
// Process the form submission and schedule the Zoom meeting
// Retrieve form inputs from $request
$startDateTime = $request->input('start_date') . ' ' . $request->input('start_time');
$topic = $request->topic;
$duration = $request->duration;
$chatId = $request->chatID;
$token = $this->getZoomAccessToken();
// Example code to create a meeting using the Zoom API
$client = new Client();
$response = $client->post('https://api.zoom.us/v2/users/me/meetings', [
'headers' => [
'Authorization' => 'Bearer ' . $token,
'Content-Type' => 'application/json',
],
'json' => [
'topic' => $request->input('topic'),
'start_time' => date('Y-m-d\TH:i:s', strtotime($startDateTime)),
'duration' => $request->input('duration'),
'settings' => [
'host_video' => true,
'participant_video' => true,
'mute_upon_entry' => true,
],
'agenda' => $topic,
'recurrence' => [
'type' => 1,
'repeat_interval' => 1,
'weekly_days' => '1',
'end_times' => $duration,
],
'settings' => [
'host_video' => true,
'participant_video' => true,
],
],
]);
$responseData = json_decode($response->getBody(), true);
if ($response->getStatusCode() === 201) {
// Meeting created successfully
$joinUrl = $responseData['join_url'];
$meetingId = $responseData['id'];
$passcode = $responseData['password'];
// Return a response or redirect as needed
return redirect()->back()->with('success', 'Zoom meeting scheduled successfully!');
} else {
// Meeting creation failed, handle the error scenario
return redirect()->back()->with('error', 'Failed to schedule Zoom meeting.');
}
// Return a response or redirect as needed
}
private function getZoomAccessToken()
{
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://zoom.us/oauth/token?grant_type=account_credentials&account_id='.env('ZOOM_ACCOUNT_ID'),
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_HTTPHEADER => array(
'Authorization:'. 'Basic ' . base64_encode(env('ZOOM_CLIENT_ID') . ':' . env('ZOOM_CLIENT_SECRET')),
'Cookie: _zm_mtk_guid=d23df74c2a9d4765b5a94142dc2d87d0'
),
));
$response = curl_exec($curl);
curl_close($curl);
$responseData = json_decode($response, true);
return $responseData['access_token'];
}
}
Step 6: Create a Blade View for Scheduling Form
Create a Blade view file where users can input the meeting details and schedule the meeting. For example, you can create schedule-meeting.blade.php
in the resources/views
directory:
<form action="{{ route('zoom.schedule') }}" method="post">
@csrf
<label for="topic">Meeting Topic:</label>
<input type="text" name="topic" required><br>
<label for="start_date">Start Date:</label>
<input type="date" name="start_date" required><br>
<label for="start_time">Start Time:</label>
<input type="time" name="start_time" required><br>
<label for="duration">Duration (in minutes):</label>
<input type="number" name="duration" required><br>
<button type="submit">Schedule Zoom Meeting</button>
</form>
Step 7: Define Routes
In the routes/web.php
file, define a route to handle the form submission:
use App\Http\Controllers\ZoomMeetingController;
Route::post('/schedule-zoom-meeting', [ZoomMeetingController::class, 'scheduleMeeting'])->name('zoom.schedule');
Step 8: Run the Application
Now, run your Laravel application using the php artisan serve
command and visit the page where you have the scheduling form. Fill in the meeting details and click the “Schedule Zoom Meeting” button. The meeting will be scheduled using the Zoom API, and the join URL will be returned.
Read More
1 thought on “Laravel shedule zoom metting in 2023 using new Server-to-Server OAuth or OAuth app types”
Comments are closed.