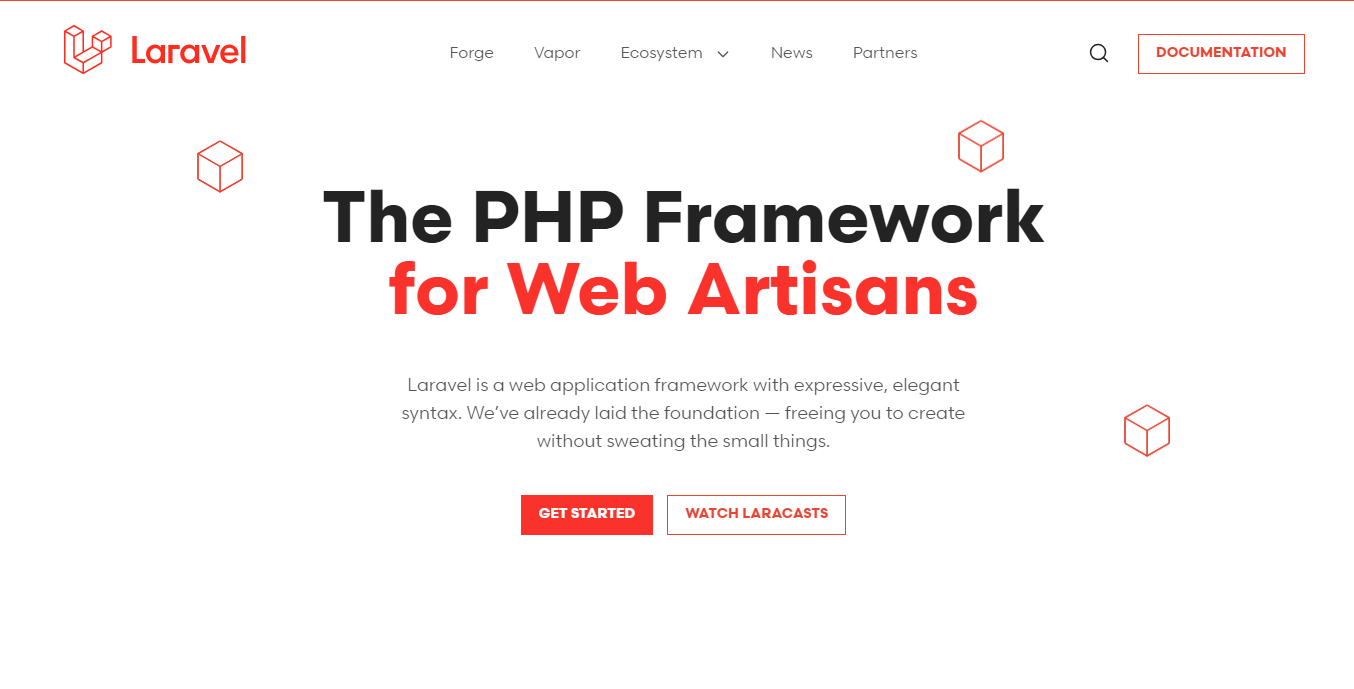
laravel localization
In this tutorial, we’ll use the built-in Web Speech API provided by modern web browsers. The Web Speech API allows us to perform text-to-speech without relying on external libraries, making it a lightweight and convenient solution.
Step 1: Install Laravel
Begin by installing a new Laravel project. Open your terminal or command prompt and run the following command to create a new Laravel project:
composer create-project --prefer-dist laravel/laravel text-to-speech-app
cd text-to-speech-app
Step 2: Create a Text-to-Speech View
Next, create a Blade view where users can input text and listen to the speech output. In the resources/views
directory, create a new Blade file named text_to_speech.blade.php
.
<!-- resources/views/text_to_speech.blade.php -->
<!DOCTYPE html>
<html>
<head>
<title>Text to Speech</title>
</head>
<body>
<h1>Text to Speech Conversion</h1>
<input type="text" id="textInput" placeholder="Enter text to speak">
<button id="speakButton">Speak</button>
<script>
// JavaScript code will be added later
</script>
</body>
</html>
This view provides a user interface with an input field where users can enter text and a “Speak” button to trigger the text-to-speech conversion.
Step 3: Implement JavaScript for Text-to-Speech
In the same text_to_speech.blade.php
file, add JavaScript code to handle text-to-speech functionality using the Web Speech API.
<!-- resources/views/text_to_speech.blade.php -->
<script>
document.getElementById('speakButton').addEventListener('click', function() {
const text = document.getElementById('textInput').value;
const sentences = text.match(/[^\.!\?]+[\.!\?]+/g); // Split text into sentences
if (sentences && sentences.length > 0) {
speak(sentences);
} else {
alert('Please enter some text to speak.');
}
});
function speak(sentences) {
if ('speechSynthesis' in window) {
const speechQueue = [];
sentences.forEach(function(sentence) {
const speech = new SpeechSynthesisUtterance();
speech.text = sentence;
speechQueue.push(speech);
});
playSpeechQueue(speechQueue);
} else {
alert('Text-to-speech is not supported in this browser.');
}
}
function playSpeechQueue(queue) {
if (queue.length > 0) {
const speech = queue.shift();
speech.onend = function() {
playSpeechQueue(queue); // Play the next sentence after the current one ends
};
window.speechSynthesis.speak(speech);
}
}
</script>
This JavaScript code handles the text-to-speech functionality. When the user clicks the “Speak” button, the entered text will be split into sentences, and each sentence will be synthesized separately using the Web Speech API. The function playSpeechQueue
is introduced to handle the sequential playback of each sentence.
Step 4: Add a Route to Access the Text-to-Speech View
In the routes/web.php
file, add the following route definition:
// routes/web.php
Route::get('/text-to-speech', function () {
return view('text_to_speech');
});
This route specifies that when a user accesses the /text-to-speech
URL, it will return the text_to_speech.blade.php
view we created earlier.
Step 5: Access the Text-to-Speech Page
Start the Laravel development server by running the following command (if you haven’t already):
php artisan serve
Now, visit http://localhost:8000/text-to-speech
in your web browser to access the text-to-speech page. You can enter text in the input field and click the “Speak” button to listen to the speech output.
With the additional route defined, users can now access the text-to-speech page directly through the /text-to-speech
URL, and Laravel will serve the corresponding view.
Congratulations! You’ve successfully implemented client-side text-to-speech functionality in your Laravel application using the Web Speech API. Users can now enjoy enhanced accessibility by listening to the content in your application. This lightweight and user-friendly feature can greatly improve the overall user experience.
Read More