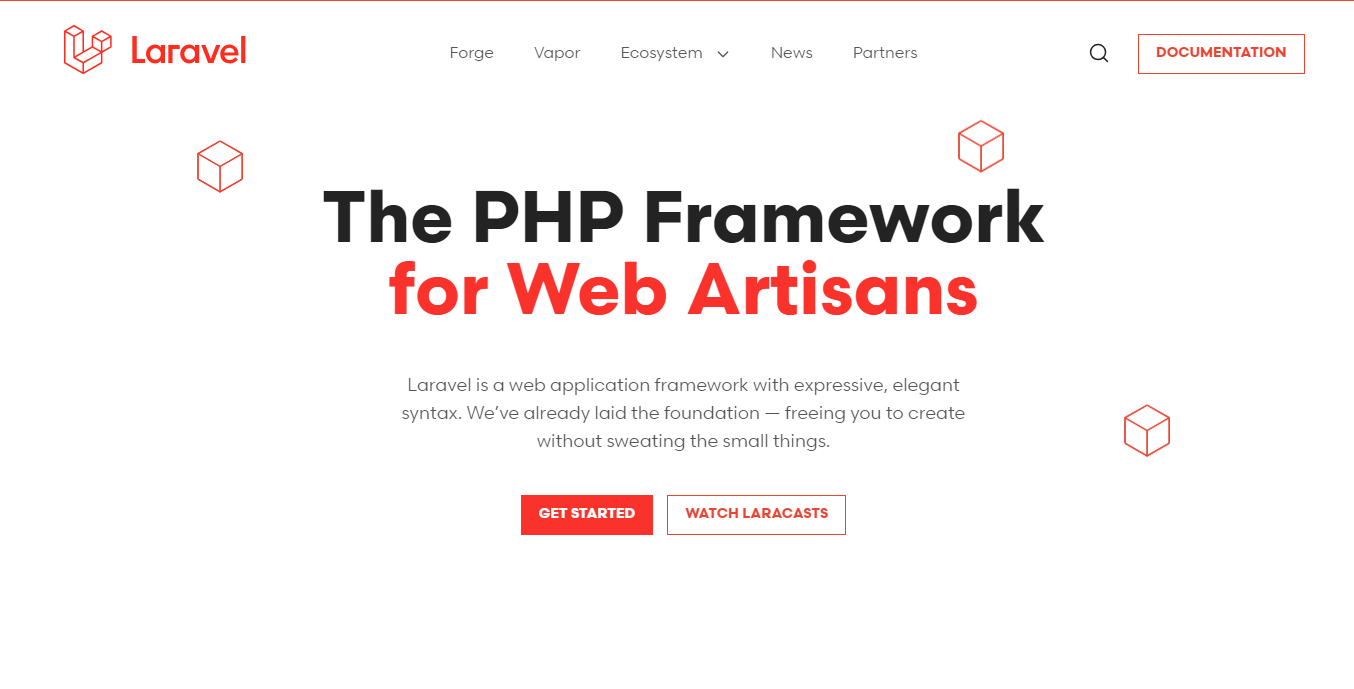
laravel localization
Laravel, the popular PHP framework, makes it easy to build robust web applications. One of its powerful features is localization, allowing developers to create multilingual applications effortlessly. This guide will walk you through the process of implementing Laravel localization, ensuring your application can cater to a global audience.
What is Localization? Localization is the process of adapting your application to different languages and regions. It involves translating user interface text and formatting dates, numbers, and other locale-specific data.
Setting Up Localization in Laravel: To get started with localization in Laravel, follow these steps:
1.Configuration: Open your config/app.php
file and set the locale
and fallback_locale
options:
'locale' => 'en',
'fallback_locale' => 'en',
2.Language Directories: Laravel stores language files in the resources/lang
directory. Create a subdirectory for each language you want to support (e.g., resources/lang/en
for English, resources/lang/es
for Spanish).
Creating Language Files: Language files contain key-value pairs for translations. Let’s create a language file for English and Spanish.
English (resources/lang/en/messages.php
):
return [
'welcome' => 'Welcome to our application',
'thank_you' => 'Thank you for using our application',
];
Spanish (resources/lang/es/messages.php
):
return [
'welcome' => 'Bienvenido a nuestra aplicación',
'thank_you' => 'Gracias por usar nuestra aplicación',
];
Switching Between Languages: To switch between languages, you can use the App::setLocale()
method. Here’s an example of how to change the locale based on user preference:
Route for Changing Language:
Route::get('lang/{locale}', function ($locale) {
if (!in_array($locale, ['en', 'es'])) {
abort(400);
}
App::setLocale($locale);
return redirect()->back();
});
Blade Template:
<a href="{{ url('lang/en') }}">English</a>
<a href="{{ url('lang/es') }}">Spanish</a>
Advanced Localization Techniques:
Pluralization: Laravel supports pluralization for languages with complex pluralization rules. Use the pipe (|
) character to define singular and plural forms in your language files.
'apples' => 'There is one apple|There are many apples',
Placeholders: You can use placeholders in your translations.
'welcome_user' => 'Welcome, :name!',
Usage:
{{ __('messages.welcome_user', ['name' => 'John']) }}
JSON Translations: For applications with many translation strings, JSON translation files are a great alternative. Create a resources/lang/en.json
file:
{
"Welcome to our application": "Welcome to our application",
"Thank you for using our application": "Thank you for using our application"
}
Conclusion: Implementing localization in Laravel is straightforward and powerful. By following this guide, you can create a multilingual application that caters to a global audience, enhancing user experience and expanding your reach. With advanced techniques like pluralization and placeholders, Laravel localization offers flexibility and ease of use.
Read about laravel