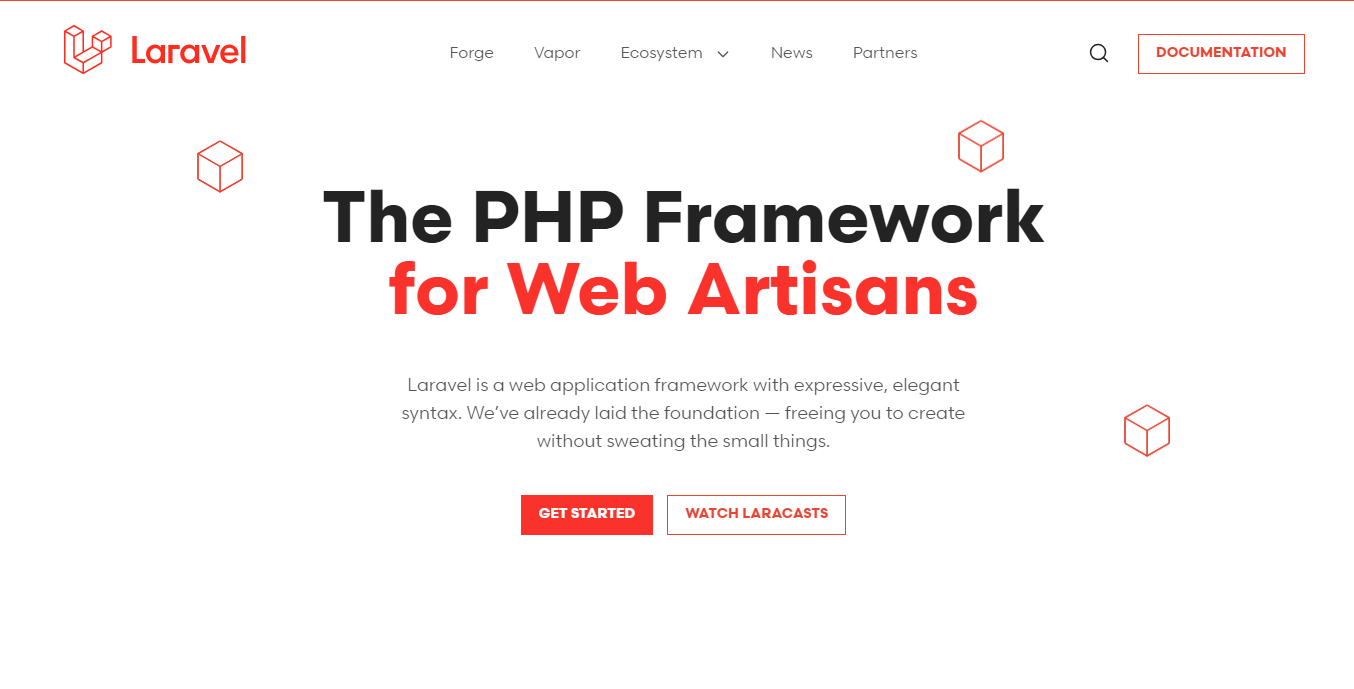
laravel localization
Learn effective techniques to debug your Laravel code, ensuring smoother development and troubleshooting processes.
Debugging in Laravel: An Overview
Before diving into the specifics, understand that debugging is the process of identifying and fixing errors in your code. It’s crucial for maintaining the quality and functionality of your Laravel applications.
Part 1: Debugging Tools and Strategies
Step1: Utilize Laravel Debugging Tools
Laravel provides built-in tools like error pages, logging, and stack traces. These tools offer valuable insights when something goes wrong, helping you pinpoint the root cause of errors. Additionally, the dd()
method allows you to dump and die, providing a snapshot of variables and their values at a specific point in your code.
Step2: Leverage Artisan Commands
Artisan commands are powerful tools for debugging. For instance, tinker
allows you to interactively explore your application, route:list
displays all registered routes, and event:listen
helps track events and listeners.
Step3: Implement Debugging Packages
Third-party packages like Debugbar
and Clockwork
extend Laravel’s debugging capabilities. They provide visual representations of queries, performance metrics, and more, aiding in comprehensive troubleshooting.
Part 2: Troubleshooting Common Scenarios
Step1: Handling Database Queries
Database queries are a common source of issues. Learn how to use Laravel’s query builder to inspect queries and identify inefficiencies like N+1 queries, optimizing database interactions.
Step2: Debugging Views and Templates
Blade templates are integral to Laravel’s UI. Discover techniques to inspect and debug templates, ensuring correct data rendering and resolving view-related errors.
Step3: Uncover Logic and Routing Issues
Troubleshoot logic and routing problems that affect your application’s behavior. Identify and resolve issues related to controllers, routes, and middleware, ensuring seamless user experiences.
Part 3: Advanced Debugging Techniques
Step1: Debugging API Requests
API debugging requires specific tools. Learn how to use tools like Postman and Laravel’s built-in features to analyze API requests and responses, ensuring accurate communication.
Step2: Debugging with Xdebug
Xdebug is a powerful PHP extension for in-depth debugging. Configure it with Laravel and your preferred IDE to step through code, inspect variables, and identify errors systematically.
Conclusion
Congratulations! By exploring this comprehensive guide, you’ve unlocked the art of debugging in Laravel. Equipped with a range of strategies and tools, including the indispensable dd()
method, you can confidently tackle issues head-on. Embrace debugging as a crucial part of your development workflow, ensuring seamless code execution and faster troubleshooting. With these skills, your coding and debugging journey is bound for success. Happy coding and debugging!
Read More
3 thoughts on “Debugging Laravel Code: A Comprehensive Guide”
Comments are closed.