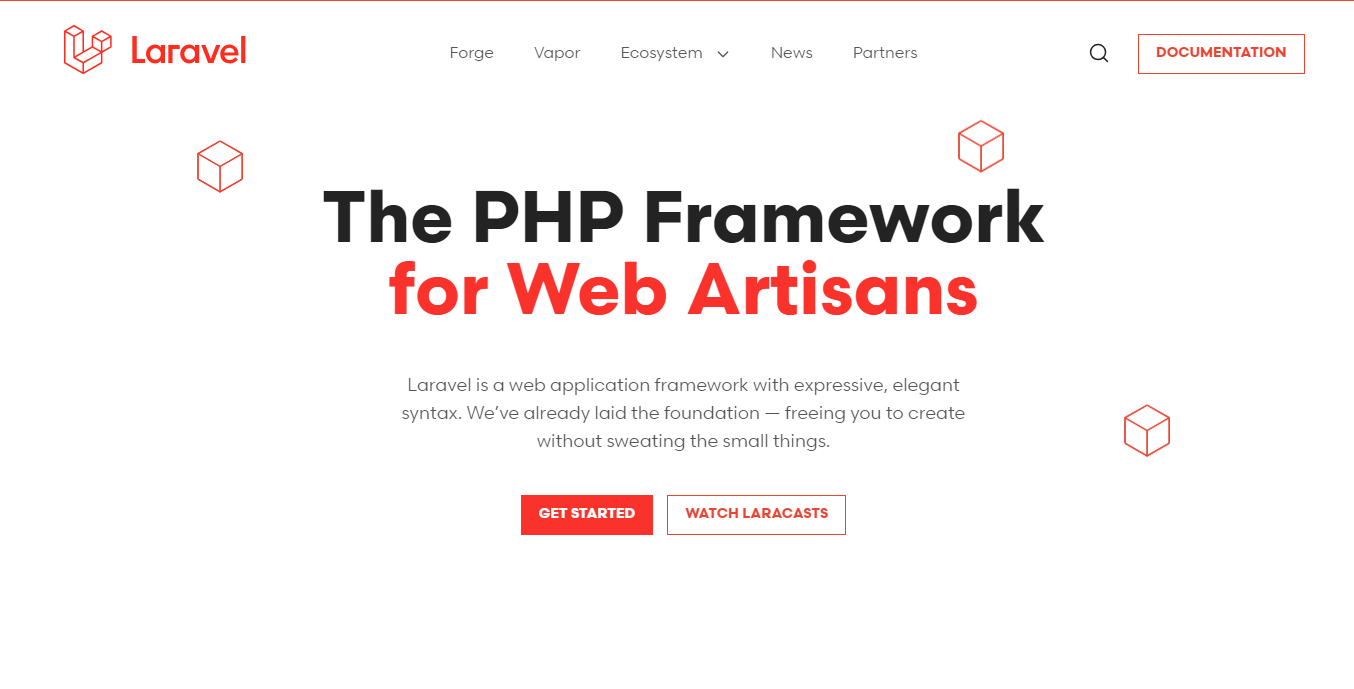
laravel localization
In this tutorial, you’ll learn how to convert a string to uppercase in Laravel using both a controller method and a Blade template.
Part 1: Converting String to Uppercase in Laravel Controller
Step1: Create a New Controller
- Open your Laravel project’s terminal.
- Use the
php artisan make:controller
command to create a new controller. For instance, create an “UppercaseController”:
php artisan make:controller UppercaseController
Step2: Implement Uppercase Conversion
- Open the newly created
UppercaseController.php
file in theapp/Http/Controllers
directory. - In a controller method, use the
Str::upper()
function to convert a string to uppercase:
use Illuminate\Support\Str;
// ...
public function toUppercase($inputString)
{
$uppercaseString = Str::upper($inputString);
return view('uppercase', ['inputString' => $inputString, 'uppercaseString' => $uppercaseString]);
}
Step3: Define Route
- Open the
routes/web.php
file. - Define a route that uses the
toUppercase
method in theUppercaseController
:
Route::get('/uppercase/{input}', 'UppercaseController@toUppercase');
Part 2: Displaying Uppercase String in Laravel Blade Template
Step1: Create a Blade View
- Navigate to your Laravel project’s
resources/views
directory. - Create a new Blade view file. For instance, name it “uppercase.blade.php”.
Step2: Display Uppercase String in Blade Template
- Open the “uppercase.blade.php” file.
- Inside the Blade template, display the uppercase string passed from the controller:
<!DOCTYPE html>
<html>
<head>
<title>String to Uppercase Conversion</title>
</head>
<body>
<p>Uppercase String Controller: {{ $uppercaseString}}</p>
<p>Uppercase String Blade: {{ Str::upper($inputString) }}</p>
</body>
</html>
Conclusion
Congratulations on mastering string-to-uppercase conversion in Laravel using controller methods and Blade templates. This versatile technique enhances your ability to manipulate text throughout your Laravel applications. Explore and integrate this method to efficiently transform strings to Uppercase. Happy coding!
Read More
2 thoughts on “Convert String to Uppercase in Laravel”
Comments are closed.