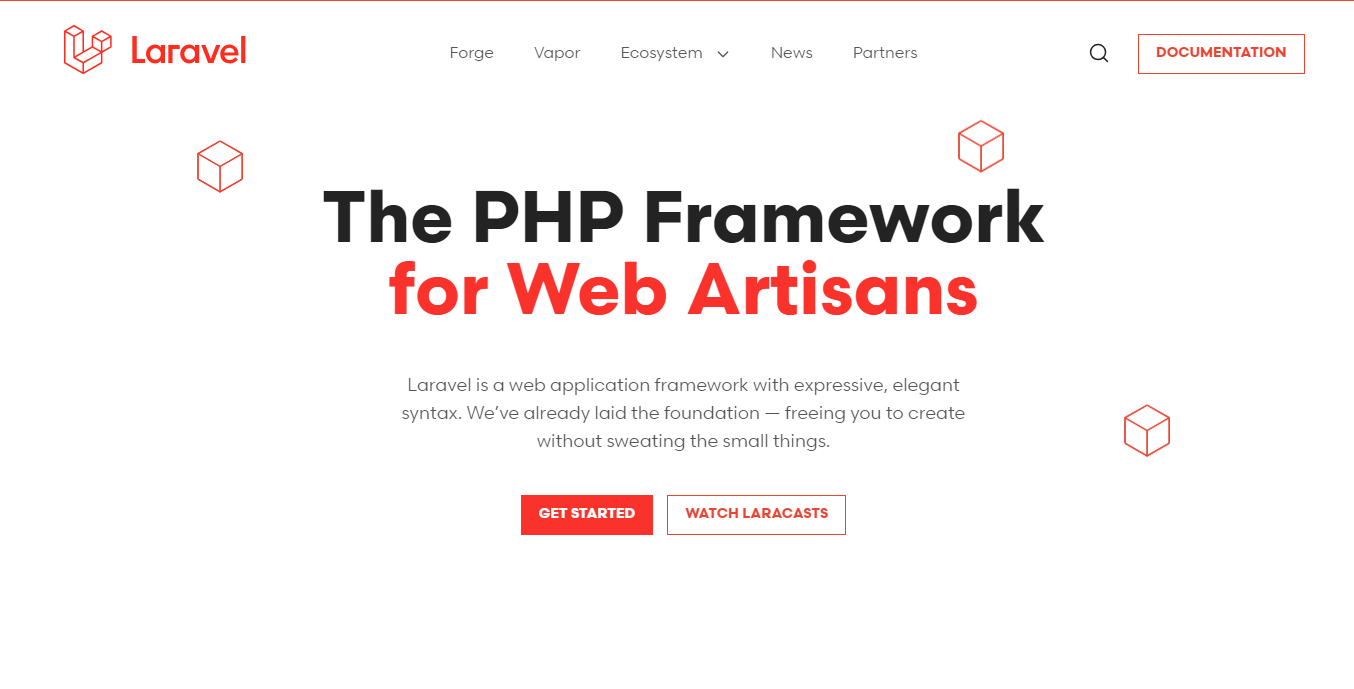
laravel localization
In this tutorial, you’ll learn how to convert a string to lowercase in Laravel using both a controller method and a Blade template.
Part 1: Converting String to Lowercase in Laravel Controller
Step1: Create a New Controller
- Open your Laravel project’s terminal.
- Use the
php artisan make:controller
command to create a new controller. For instance, create a “LowercaseController”:
php artisan make:controller LowercaseController
Step2: Implement Lowercase Conversion
- Open the newly created
LowercaseController.php
file in theapp/Http/Controllers
directory. - In a controller method, use the
Str::lower()
function to convert a string to lowercase:
use Illuminate\Support\Str;
// ...
public function toLowercase($inputString)
{
$lowercaseString = Str::lower($inputString);
return view('lowercase', ['inputString' => $inputString, 'lowercaseString' => $lowercaseString]);
}
Step3: Define Route
- Open the
routes/web.php
file. - Define a route that uses the
toLowercase
method in theLowercaseController
:
Route::get('/lowercase/{input}', 'LowercaseController@toLowercase');
Part 2: Displaying Lowercase String in Laravel Blade Template
Step1: Create a Blade View
- Navigate to your Laravel project’s
resources/views
directory. - Create a new Blade view file. For instance, name it “lowercase.blade.php”.
Step2: Display Lowercase String in Blade Template
- Open the “lowercase.blade.php” file.
- Inside the Blade template, display the lowercase string passed from the controller:
<!DOCTYPE html>
<html>
<head>
<title>String to Lowercase Conversion</title>
</head>
<body>
<p>Original String: {{ $inputString }}</p>
<p>Lowercase String: {{ Str::lower($lowercaseString) }}</p>
</body>
</html>
Conclusion
Congratulations on mastering string-to-lowercase conversion in Laravel using controller methods and Blade templates. This versatile technique enhances your ability to manipulate text throughout your Laravel applications. Explore and integrate this method to efficiently transform strings to lowercase. Happy coding!
Read More
1 thought on “Convert String to Lowercase in Laravel”
Comments are closed.