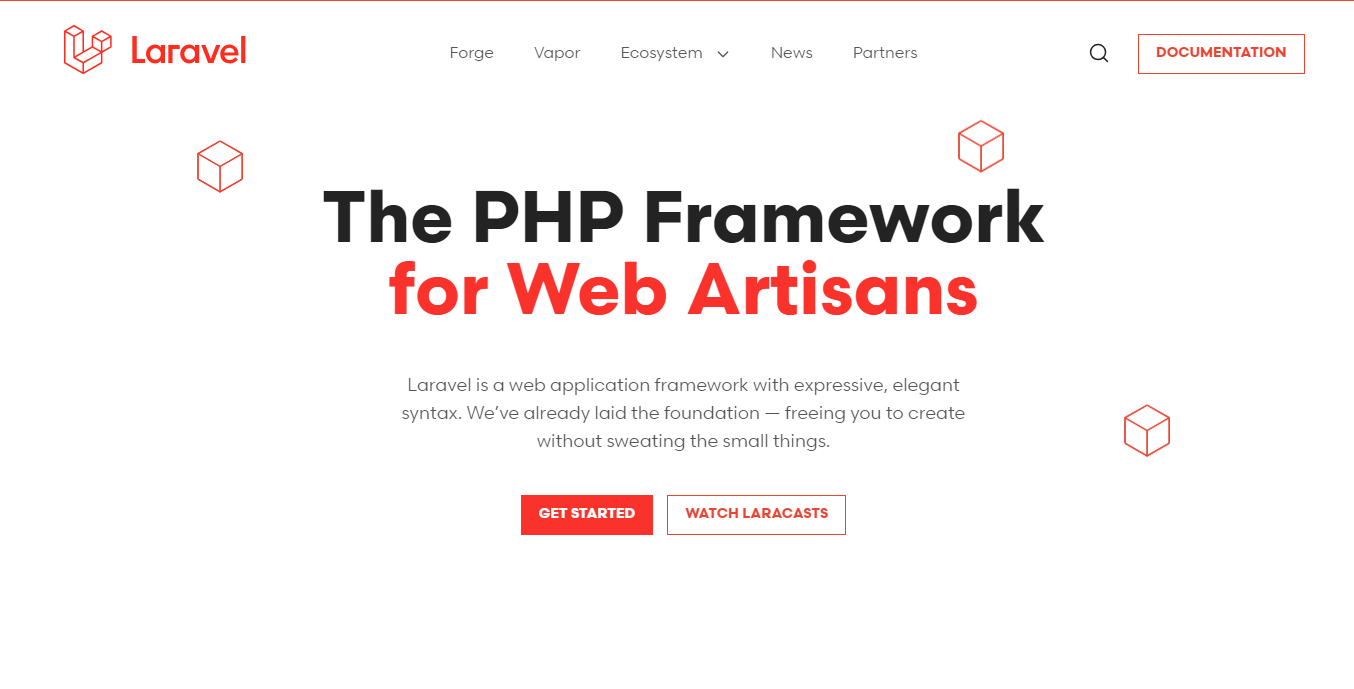
laravel localization
In this tutorial, you’ll learn how to convert a string to CamelCase in Laravel using both a controller method and a Blade template.
Part 1: Converting String to CamelCase in Laravel Controller
Step1: Create a New Controller
- Open your Laravel project’s terminal.
- Use the
php artisan make:controller
command to create a new controller. For instance, create a “CamelCaseController”:
php artisan make:controller CamelCaseController
Step2: Implement CamelCase Conversion
- Open the newly created
CamelCaseController.php
file in theapp/Http/Controllers
directory. - In a controller method, use the
Str::studly()
function to convert a string to CamelCase:
use Illuminate\Support\Str;
// ...
public function toCamelCase($inputString)
{
$camelCaseString = Str::studly($inputString);
return view('camel-case', ['inputString' => $inputString, 'camelCaseString' => $camelCaseString]);
}
Step3: Define Route
- Open the
routes/web.php
file. - Define a route that uses the
toCamelCase
method in theCamelCaseController
:
Route::get('/camel-case/{input}', 'CamelCaseController@toCamelCase');
Part 2: Displaying CamelCase String in Laravel Blade Template
Step1: Create a Blade View
- Navigate to your Laravel project’s
resources/views
directory. - Create a new Blade view file. For instance, name it “camel-case.blade.php”.
Step2: Display CamelCase String in Blade Template
- Open the “camel-case.blade.php” file.
- Inside the Blade template, display the CamelCase string passed from the controller:
<!DOCTYPE html>
<html>
<head>
<title>String to CamelCase Conversion</title>
</head>
<body>
<p>Original String: {{ $inputString }}</p>
<p>CamelCase String: {{ Str::studly($inputString) }}</p>
</body>
</html>
Conclusion
Congratulations! You’ve successfully learned how to convert a string to CamelCase in Laravel using both a controller method and a Blade template. This technique can be applied to transform strings for various purposes in your Laravel application.
Feel free to explore further and use this method wherever you need to convert strings to CamelCase. Happy coding!
Read More
1 thought on “Convert String to CamelCase in Laravel”
Comments are closed.