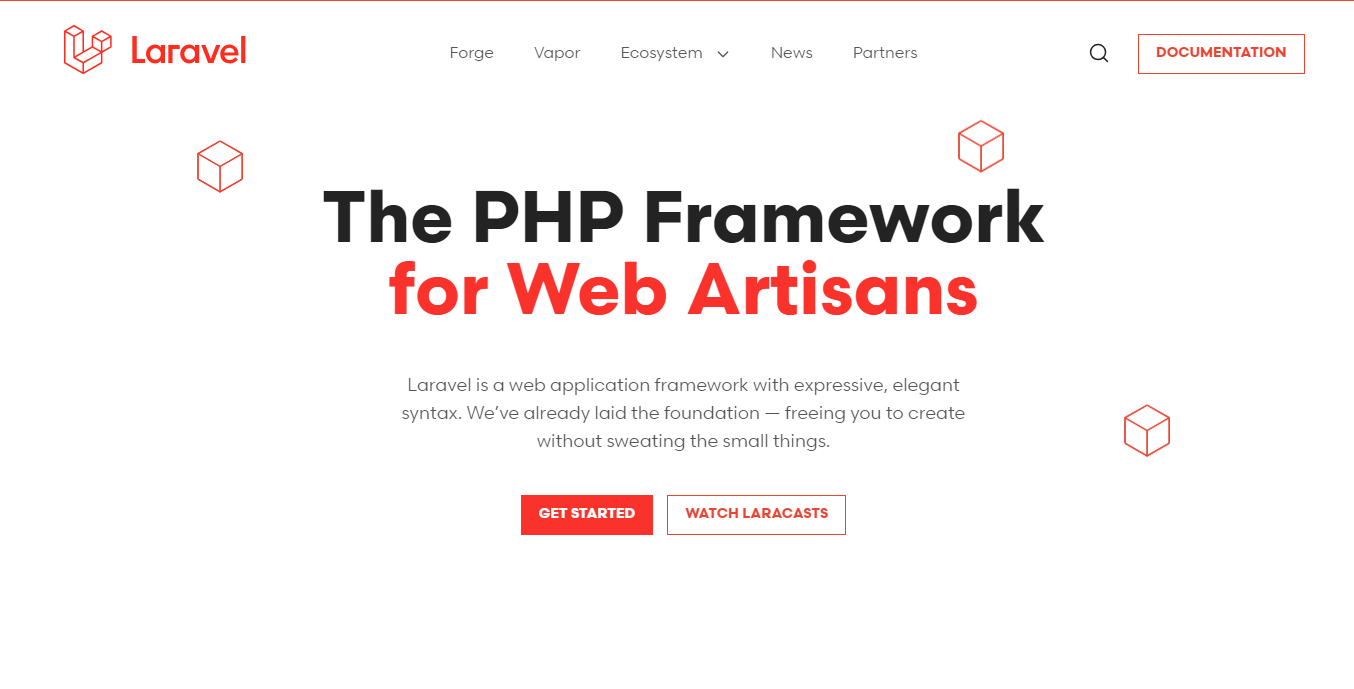
laravel localization
Cross-Site Request Forgery (CSRF) is a security vulnerability that allows an attacker to trick users into unknowingly submitting requests to a website. To prevent CSRF attacks in Laravel applications, you should use CSRF tokens and follow best practices when working with AJAX requests. Here are the best practices for CSRF token management in AJAX requests in Laravel:
Include CSRF Token in your Layout
In your main layout or master template, include the CSRF token in the meta tag or a data attribute. Laravel provides a convenient csrf
Blade directive that you can use to generate the CSRF token.
<meta name="csrf-token" content="{{ csrf_token() }}">
Configure AJAX Headers
In your JavaScript code, configure the default headers for all AJAX requests to include the CSRF token. You can use the X-CSRF-TOKEN
header to send the token along with the request.
// Set the CSRF token for all AJAX requests
$.ajaxSetup({
headers: {
'X-CSRF-TOKEN': $('meta[name="csrf-token"]').attr('content')
}
});
Use CSRF Token in AJAX Requests
When making AJAX requests, ensure that the CSRF token is included in the request headers. For example, with jQuery:
$.ajax({
url: '/your-ajax-endpoint',
type: 'POST',
data: { /* your data here */ },
success: function(response) {
// Handle success
},
error: function(error) {
// Handle error
}
});
Protect Sensitive Routes and Actions
In Laravel, you can use the csrf
middleware to automatically verify the CSRF token on all POST, PUT, DELETE, and PATCH routes. Apply this middleware to any route that modifies data on the server to ensure proper CSRF protection.
Route::middleware('csrf')->group(function () {
// Your protected routes here
});
Consider Using Laravel’s @csrf
Directive
Instead of manually adding the CSRF token meta tag, you can use Laravel’s @csrf
Blade directive. Place this directive inside your form tags, and it will automatically generate the CSRF token field
<form method="POST" action="/your-form-action">
@csrf
<!-- Rest of the form -->
</form>
By following these best practices, you can ensure that your Laravel application is well-protected against CSRF attacks when using AJAX requests. It’s essential to maintain strong security measures to safeguard your application and its users’ data.
Read More
2 thoughts on “Best Practice in CSRF Token Management for AJAX in Laravel”
Comments are closed.