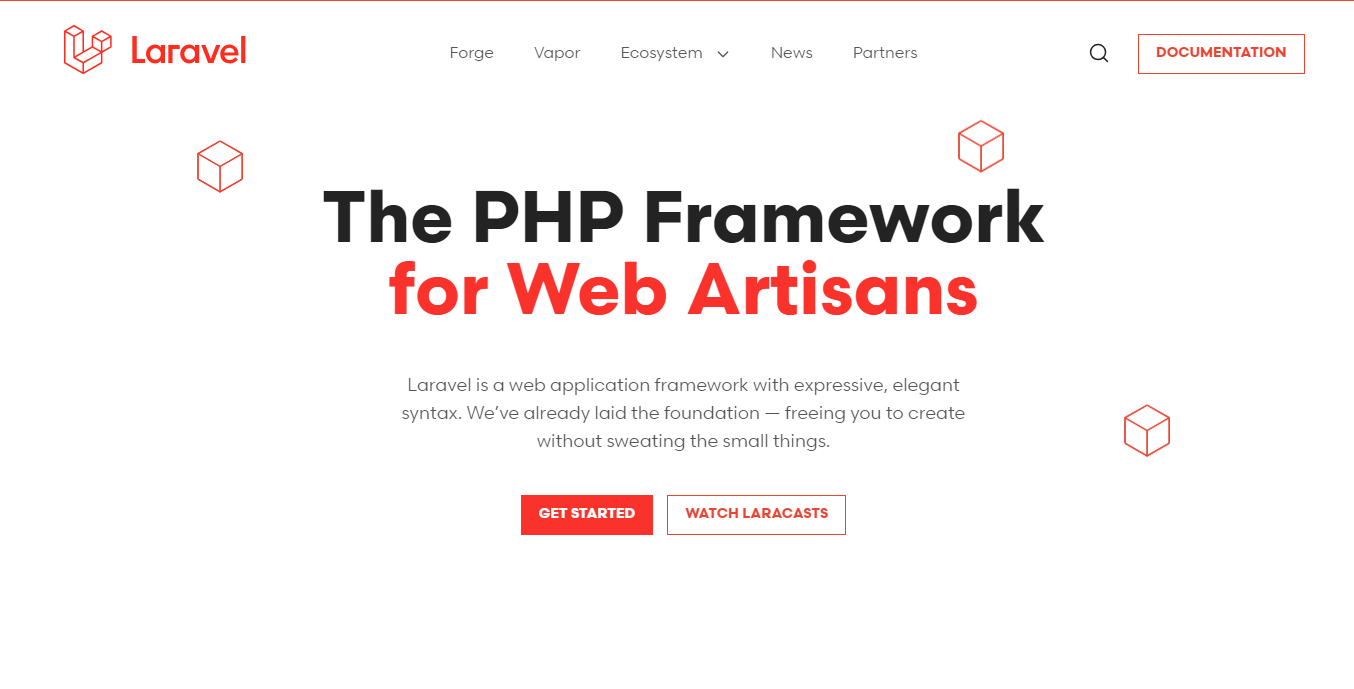
laravel localization
To send messages to a Telegram group using Laravel, you can utilize the Telegram Bot API and the Laravel HTTP client. Here’s a step-by-step guide:
Step 1: Create a Blade file with a message box
Create a new Blade file, for example, send_message.blade.php
in the resources/views
directory of your Laravel project. Add the following code to create a simple form with a message box where users can type the message they want to send to the Telegram group.
<!-- resources/views/send_message.blade.php -->
@extends('layouts.app')
@section('content')
<div class="container">
<h1>Send Message to Telegram Group</h1>
<form method="POST" action="{{ route('sendMessage') }}">
@csrf
<div class="form-group">
<label for="message">Message:</label>
<textarea class="form-control" id="message" name="message" rows="3"></textarea>
</div>
<button type="submit" class="btn btn-primary">Send Message</button>
</form>
</div>
@endsection
Step 2: Create a route to send the message
Add a new route in your routes/web.php
file that points to the controller function responsible for sending the message.
// routes/web.php
use App\Http\Controllers\TelegramController;
Route::get('/send-message', [TelegramController::class, 'showSendMessageForm'])->name('showSendMessageForm');
Route::post('/send-message', [TelegramController::class, 'sendMessage'])->name('sendMessage');
Step 3: Create a controller to handle the message sending
Create a new controller named TelegramController
that will handle the message sending logic. Run the following command to generate the controller:
php artisan make:controller TelegramController
Then, update the TelegramController
with the following code:
// app/Http/Controllers/TelegramController.php namespace App\Http\Controllers; use Illuminate\Http\Request; use Illuminate\Support\Facades\Http; class TelegramController extends Controller { // Show the form to send a message public function showSendMessageForm() { return view('send_message'); } // Get the group chat ID where the bot is added public function getGroupChatId() { $response = Http::get("https://api.telegram.org/botYOUR_BOT_TOKEN/getUpdates"); $data = $response->json(); if (isset($data['result']) && !empty($data['result'])) { // The latest update should contain the group chat ID $latestUpdate = end($data['result']); $groupChatId = $latestUpdate['message']['chat']['id']; return $groupChatId; } return null; // No group chat ID found } // Send the message to the Telegram group public function sendMessage(Request $request) { $message = $request->input('message'); $groupChatId = $this->getGroupChatId(); if (!$groupChatId) { return redirect()->back()->with('error', 'Group chat ID not found.'); } // Send the message to the Telegram group Http::post("https://api.telegram.org/botYOUR_BOT_TOKEN/sendMessage", [ 'chat_id' => $groupChatId, 'text' => $message, ]); return redirect()->back()->with('success', 'Message sent successfully!'); } }
Replace YOUR_BOT_TOKEN
with the actual bot token obtained from the BotFather. The getGroupChatId()
function will fetch the latest updates from the Telegram API and extract the group chat ID from the message object.
Step 5: Display the form to send the message
Now, when you visit the URL /send-message
, you should see the form to send a message to the Telegram group. Fill in the message and click the “Send Message” button. The message will be sent to the specified Telegram group using the Telegram Bot API.
Please make sure to handle form validation and error handling as per your requirements in the controller and the Blade file.
Read More
1 thought on “Laravel Send Telegram Notifications To Telegram Group via Telegram Bot”
Comments are closed.